ES2017 introduced JavaScript Async/Await which is a wrapper around Promises. Async/Await is used to consume promise-based APIs in the modern and simplest way.
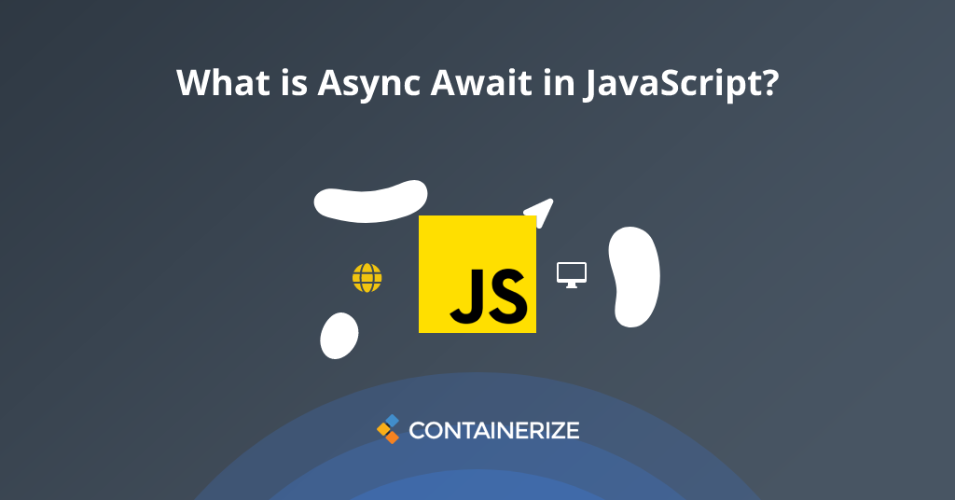
Overview
We covered JavaScript Promises in our previous blog post and you must visit if you want to get a clear understanding of the concept behind JavaScript Promises. In this series of JavaScript tutorials, we are here with another JavaScript guide that will demonstrate the modern features of JavaScript Async/Await. This blog post is for those who have prior knowledge of JavaScript Promises. Further, we will try to cover What is Async/Await in JavaScript, and when we need to use Async/Await. In addition, we will also cover handling errors with Async/Await. So, give a thorough read to this JavaScript Async/Await tutorial to mark this concept checked.
We will go through the following sections in this JavaScript Async Await tutorial:
- What is Async/Await in JavaScript | Async Await Syntax
- When to use Async/Await?
- Error handling with Async/Await
What is Async/Await in JavaScript | Async Await Syntax
ECMAScript 2017 released new features of JavaScript and Async/Await is one of the most widely used among these features. Async/await is built on top of Promises and is actually a modern way to handle JavaScript Promises.
Let’s see how to use Async/Await keywords in the JavaScript code:
async function getValues() {
let promise = new Promise(function(resolve, reject) {
// processing..
});
let data = await promise; // waits until the promise fulfills
return data;
}
getValues().then(function(){
console.log(data); // results printed.
})
Well, the async keyword at the start of the function implies that this function will return a promise. So, await keyword is always used inside the body of the async function to pause the execution of the function until the promise resolves. The important thing to note here is that you cannot use await keyword inside the body of a regular function. However, you can use await only with asynchronous functions declared with the async keyword.
When to use Async/Await?
So far, you have the answer to what is Async Await in JavaScript. Since this is only a wrapper around the traditional JavaScript promises so you better use this new way to create and handle promises in a more cleaner and readable way. In addition, you can get rid of multiple promise.then() calls using async/await which eventually makes source code more manageable and maintainable. The await keyword encapsulates the .then() statement into a single line.
Error handling with Async/Await
Error handling is a critical task when it comes to enterprise-level software development. There are multiple ways to catch errors in Async/Await approach.
Try Catch Async Await JavaScript: Nothing new with this error handling approach. However, you can use a try/catch block inside an async function as shown in the code snippet below:
async function getValues() {
try{
let promise = new Promise(function(resolve, reject) {
// processing..
});
let data = await promise; // waits until the promise fulfills
return data;
} catch(err){
// you may handle errors the way you want..
}
}
Using the try/catch block, you can catch the exceptions inside the body of an async function.
Whereas, there is another approach to handling errors in which you can append the .catch() block at the end of the function call. Since async/await returns a promise, so you catch the errors in the .catch() block.
async function getValues() {
let promise = new Promise(function(resolve, reject) {
// processing..
});
let data = await promise; // waits until the promise fulfills
return data;
}
getValues().catch(err){
// catch the errors
}
Conclusion
We are ending this JavaScript async await tutorial. Hope you have a better understanding of What is Async Await in JavaScript. In addition, we have gone through JavaScript async await syntax that makes source code less complex. This JavaScript feature is being widely used due to its rich usage and performance. In the coming days, we will write further on JavaScript features and concepts so that you can gain a strong command over JS concepts. Moreover, there are some other interesting articles mentioned in the “See Also” section.
Connect with us
Lastly, containerize.com offers ongoing JavaScript tutorials on various exciting topics. You can stay updated by following us on our social media platforms, including Facebook, LinkedIn, and Twitter.
Ask a Question
You can let us know about your questions or queries on our forum.
FAQs
What is async await in JavaScript with example?
You may visit this link to understand the concept of async await.
What is async await vs promise?
- Async/await makes source code more clearer and readable as compared to promises in which promise chaining can make source code a mess.
- Error handling is pretty similar in both approaches.
- Debugging is less difficult in Async Await.
- Promises maintain the states such as pending, resolved, or rejected. Whereas, async/await is either resolved or rejected.
See Also
- What is Promise in JavaScript? | JavaScript Tutorial
- What is Serverless Computing? | Serverless Architecture
- What is Multitenancy? | Why a Multi-Tenant Approach?
- What is Generative AI | Generative Artificial Intelligence
- How to Integrate ChatGPT with Google Sheets
- How to use ChatGPT in VSCode | The VSCode Extension Code GPT
- What is OpenAI Chatbot GPT-3 | ChatGPT an AI Revolution
- An Introduction to Artificial Intelligence | What is AI?