Developers show positive intent in learning JavaScript design patterns. JS design patterns give your source code a design to make it efficient & maintainable.
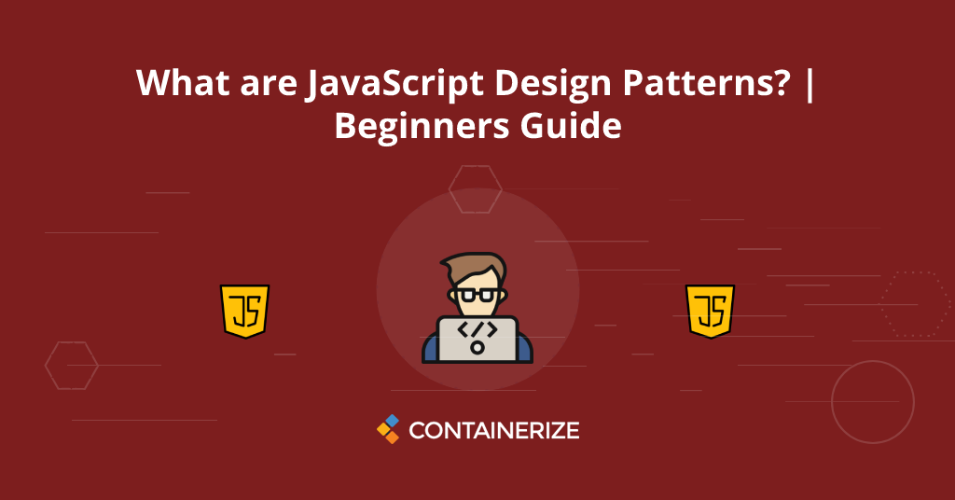
Overview
A large number of JavaScript programmers are unclear about JavaScript design patterns and this gap should be filled. In this series of JavaScript tutorials, we are writing this blog post that will cover the most widely used JavaScript design patterns such as the Module design pattern, Observer pattern, and more. In addition, we will try to demonstrate using code examples so that there is no ambiguity left in your mind. This topic is very important if you are pursuing a career in JavaScript. Therefore, stay connected throughout this article, and by the end of this blog post, you should have a strong understanding of JS design patterns.
The following points shall be covered in this JavaScript beginner’s guide:
- What are JavaScript design patterns?
- Module design pattern
- Factory pattern JavaScript
- Singleton pattern JavaScript
- Observer pattern JavaScript
What are JavaScript design patterns?
JavaScript design patterns are solutions in the form of templates that provide efficient solutions to the common problems that occur repeatedly in writing source code. In more simple words, design patterns are pre-defined styles/orders to write maintainable, and manageable JavaSciprt code. In small organizations, most developers don’t pay attention to design patterns but it becomes a critical subject when you are building large-scale business software. Basically, there are a number of developers working for enterprise-level software that have their own style of writing code. Therefore, you opt for a design pattern to prevent repeating issues, build reusable code blocks, and speed up app development.
Module design pattern
This is the most common and go-to design pattern that developers opt for. As per this design pattern, the application code blocks are put separately from each other in form of modules. This JavaScript design pattern provides isolation and encapsulation. In addition, you can make public/private functions and attributes that you cannot access from outside of the module. Above all, modules in the modular design pattern always return an object. You can use this design pattern in case you are building an enterprise-level rich featured business application. So, you can build a separate module for every feature to bring independency.
The following code snippet demonstrates the modular design pattern:
const student = (function () {
// Private
let name = "mustafa";
let program = "computer science";
const getStudentName = () => name;
const getStudentProgram = () => program;
// Public
return {
name,
program,
getName: () => getStudentName(),
getStudentProgram: () => getStudentProgram(),
};
})();
student.name;
student.program;
student.getStudentProgram();
Factory pattern JavaScript
As per this design pattern, you create objects using an interface rather than calling the constructor with the new operator. In fact, we specify a type of object and the factory creates an instance and sends us back for use. In addition, this JavaScript design pattern gives complete control over object creation. Further, you can choose this design pattern for handling simple and complex objects and it is also wise to use the Factory pattern for decoupling. Let’s write a code snippet for further exploration.
// sandwich.js
const Sandwich = function({name }) {
this.name = name || "";
};
module.exports = Sandwich;
// burger.js
const Burger = function({name }) {
this.name = name || "";
};
module.exports = Burger;
// gadFoodFactory.js
const Sandwich = require("./sandwich");
const Burger = require("./burger");
const food = { Burger, Sandwich };
module.exports = {
createFood(type, attributes) {
const GadFoodType = food[type];
return new GadFoodType(attributes);
}
};
// index.js
const gadFoodFactory = require("./gadFoodFactory");
const mySandwich = gadFoodFactory.createFood("Sandwich", {
name: "Chicken"
});
const myBurger = gadFoodFactory.createFood("Burger", {
name: "Grilled",
});
console.log(mySandwich);
console.log(myBurger);
Singleton pattern JavaScript
This design pattern is very popular that restricts the instantiation of a class to a single object. The service in the JavaScript framework Angular follows this pattern where it creates only one instance of the service and that object is accessible to all the modules. Moreover, all the client connected to the single object shares the same resources and access levels.
The demonstration of the singleton pattern is as follows:
let singletonPattern = new (function () {
let name = "mustafa";
this.printName = function() {
console.log(name);
}
})();
singletonPattern.printName();
// output: mustafa
Observer pattern JavaScript
The observer pattern is quite helpful in building scalable business apps. A notification mechanism is involved in this code pattern. Whenever there is a change, it triggers an event that notifies other dependent subscriptions. So, this pattern broadcasts the latest state to other objects across the modules. In fact, it’s all about informing the change to the subscribers automatically. The following code examples follow the observer pattern:
function ObserverFunc() {
this.observerArray = [];
}
ObserverFunc.prototype.subscribe = function (ele) {
this.observerArray.push(ele);
}
ObserverFunc.prototype.unsubscribe = function (element) {
const elementIndex = this.observerArray.indexOf(element);
if (elementIndex > -1) {
this.observerArray.splice(elementIndex, 1);
}
}
ObserverFunc.prototype.notifyAll = function (element) {
this.observerArray.forEach(function (observerElement) {
observerElement(element);
});
}
Conclusion
That’s all for the JavaScript design patterns. We hope you have enjoyed this JavaScript tutorial and learned the concepts of design patterns. We have covered the most commonly used code patterns such as module design patterns, singleton, observer, and factory design patterns. This article will give you a clear picture with the help of code snippets. In addition, there are other very relevant blog posts that you may find out in the “See Also” section below.
Connect with us
Finally, containerize.com is in a consistent process of writing JavaScript posts on new topics. You can stay in the loop by following us on our social media platforms, including Facebook, LinkedIn, and Twitter.
Ask a Question
You can let us know about your questions or queries on our forum.
FAQs
What are the most popular design patterns in JavaScript?
Module pattern, observer, factory and singleton patterns are widely used in JavaScript programming. Please follow this link to explore further.
See Also
- Learn JavaScript Basic Concepts to Help Your Career
- An Introduction to Artificial Intelligence | What is AI?
- How to Integrate ChatGPT with Google Sheets
- How to use ChatGPT in VSCode | The VSCode Extension Code GPT
- What is OpenAI Chatbot GPT-3 | ChatGPT an AI Revolution
- Google Docs Integration with ChatGPT | OpenAI GPT-3
- What are The Top 5 Open-Source AI Frameworks
- What is Generative AI | Generative Artificial Intelligence